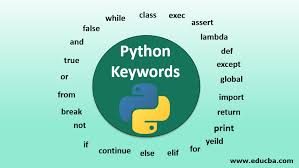
Top 10 Keywords in Python Every Developer Should Know
Python is one of the most popular programming languages today, renowned for its simplicity and readability. Whether you’re a novice or an experienced developer, understanding the core keywords in Python is essential for writing efficient and effective code. In this article, we will explore the top 10 keywords in Python that every developer should know. We’ll also touch on some of the key features of the Python programming language that make it so powerful and versatile.
1. def
The def keyword is used to define a function in Python. Functions are a fundamental aspect of Python programming, allowing you to encapsulate code into reusable blocks.
python
Copy code
def greet(name):
return f”Hello, {name}!”
2. class
The class keyword is used to define a class in Python, which is a blueprint for creating objects. Classes support object-oriented programming, one of the key features of the Python programming language.
python
Copy code
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
return f”{self.name} says woof!”
3. import
The import keyword is used to include modules in your Python script. This allows you to use external libraries and their functionalities, significantly expanding your code’s capabilities.
python
Copy code
import math
print(math.sqrt(16))
4. return
The return keyword is used in a function to send a result back to the caller. It’s a crucial part of function definitions, enabling functions to produce outputs.
python
Copy code
def add(a, b):
return a + b
5. if
The if keyword is used for conditional statements. It allows your program to execute certain pieces of code based on specific conditions, making your programs dynamic and responsive.
python
Copy code
if x > 0:
print(“x is positive”)
6. for
The for keyword is used to create loops in Python, enabling you to iterate over sequences such as lists, tuples, or strings.
python
Copy code
for i in range(5):
print(i)
7. while
The while keyword is another way to create loops in Python, which continue to execute as long as a specified condition is true.
python
Copy code
i = 0
while i < 5:
print(i)
i += 1
8. try
The try keyword is used for exception handling in Python. It allows you to handle errors gracefully and keep your program running even when it encounters unexpected situations.
python
Copy code
try:
result = 10 / 0
except ZeroDivisionError:
print(“Cannot divide by zero”)
9. with
The with keyword simplifies exception handling by encapsulating common preparation and cleanup tasks. It’s often used for managing resources like file streams.
python
Copy code
with open(‘file.txt’, ‘r’) as file:
content = file.read()
10. lambda
The lambda keyword is used to create small anonymous functions at runtime. These functions are often used for short-term, throwaway tasks.
python
Copy code
add = lambda x, y: x + y
print(add(2, 3))
Features of Python Programming Language
Understanding the keywords in Python is just one aspect of mastering this language. Here are some of the key features of the Python programming language that make it stand out:
-
Readability: Python’s syntax is designed to be readable and straightforward, which makes it easy to learn and understand.
-
Versatility: Python is a general-purpose language that can be used for web development, data analysis, artificial intelligence, scientific computing, and more.
-
Large Standard Library: Python comes with a comprehensive standard library that supports many common programming tasks, from file I/O to networking and beyond.
-
Interpreted Language: Python is an interpreted language, which means that code is executed line by line, making debugging easier.
-
Dynamic Typing: Python uses dynamic typing, meaning that you don’t have to declare variable types explicitly, which can speed up development.
-
Community and Ecosystem: Python has a large and active community, which contributes to a vast ecosystem of libraries and frameworks that extend its capabilities.
In conclusion, mastering these top 10 keywords in Python will provide a solid foundation for your programming journey. Coupled with the powerful features of the Python programming language, you’ll be well-equipped to tackle a wide range of coding challenges. Whether you’re building web applications, analyzing data, or automating tasks, Python’s keywords and features will help you write clean, efficient, and effective code.